本文概述
在Node.js中工作时遇到的问题之一是, 不断记录的消息(主要是最重要的消息)在冗长的输出中失去了详细输出。颜色在控制台上很有用, 可以使错误更加严重, 或者只是引起人们对重要错误和其他警告的注意。
在本文中, 你将学习如何在带有或不带有库的情况下, 在节点控制台中轻松显示带有颜色和样式的某些文本。
用第三方库轻松
如果你不想使自己复杂化, 那么你可能想要模块化并使用第三方库来实现你的目标。在这种情况下, 要在控制台中更改文本的颜色, 我们建议你在NPM上使用colors.js模块。此模块允许你使用可链接的方法(即” text” .bgBlue.white.underline)在node.js控制台中显示颜色和样式:
要在项目中安装colors.js模块, 请在Node.js命令提示符下执行以下命令:
npm install colors
警告:请勿将其与名称为colors.js的其他软件包混淆
在此处阅读官方Github存储库中有关库及其文档的更多信息。
文字颜色
默认方式是在String.prototype中添加一些属性(所有可用颜色作为名称), 这使得控制台中颜色的自定义变得非常容易:
var colors = require('colors');
console.log("The style of this text will be modified".black);
console.log("The style of this text will be modified".red);
console.log("The style of this text will be modified".green);
console.log("The style of this text will be modified".yellow);
console.log("The style of this text will be modified".blue);
console.log("The style of this text will be modified".magenta);
console.log("The style of this text will be modified".cyan);
console.log("The style of this text will be modified".white);
console.log("The style of this text will be modified".gray);
但是, 如果你担心扩展String.prototype, 则可以使用非原型方法使用安全模式:
var colors = require('colors/safe');
console.log(colors.black('The style of this text will be modified'));
console.log(colors.red('The style of this text will be modified'));
console.log(colors.green('The style of this text will be modified'));
console.log(colors.yellow('The style of this text will be modified'));
console.log(colors.blue('The style of this text will be modified'));
console.log(colors.magenta('The style of this text will be modified'));
console.log(colors.cyan('The style of this text will be modified'));
console.log(colors.white('The style of this text will be modified'));
console.log(colors.gray('The style of this text will be modified'));
在控制台中执行任何先前的代码片段都将产生以下结果:
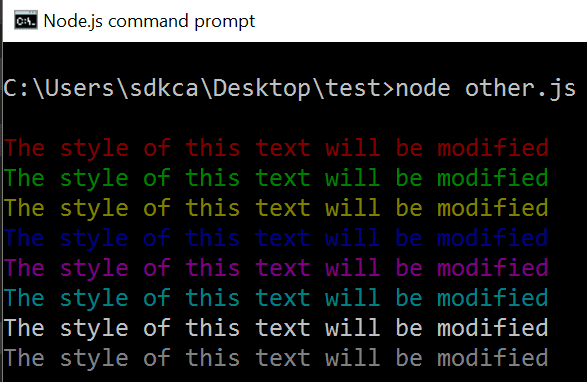
背景颜色
在控制台中向某些文本添加背景色的默认方法是在String.prototype中添加一些属性(将所有可用的颜色和前缀bg作为名称), 这使得控制台中的颜色自定义变得非常容易:
var colors = require('colors');
console.log('The style of this text will be modified'.bgBlack);
console.log('The style of this text will be modified'.bgRed);
console.log('The style of this text will be modified'.bgGreen);
console.log('The style of this text will be modified'.bgYellow);
console.log('The style of this text will be modified'.bgBlue);
console.log('The style of this text will be modified'.bgMagenta);
console.log('The style of this text will be modified'.bgCyan);
console.log('The style of this text will be modified'.bgWhite);
// Change the text color to black
console.log('The style of this text will be modified'.bgWhite.black);
// or "text".black.bgWhite
但是, 如果你担心扩展String.prototype, 则可以使用非原型方法使用安全模式:
var colors = require('colors/safe');
console.log(colors.bgBlack('The style of this text will be modified'));
console.log(colors.bgRed('The style of this text will be modified'));
console.log(colors.bgGreen('The style of this text will be modified'));
console.log(colors.bgGreen('The style of this text will be modified'));
console.log(colors.bgYellow('The style of this text will be modified'));
console.log(colors.bgBlue('The style of this text will be modified'));
console.log(colors.bgMagenta('The style of this text will be modified'));
console.log(colors.bgCyan('The style of this text will be modified'));
console.log(colors.bgWhite('The style of this text will be modified'));
// Change the text color to black
console.log(colors.bgWhite.black('The style of this text will be modified'));
// or colors.black.bgWhite("Text")
在控制台中执行任何先前的代码片段都将产生以下结果:

文字样式
Colors.js有8种可用样式:
var colors = require('colors');
console.log('The style of this text will be modified'.reset);
console.log('The style of this text will be modified'.bold);
console.log('The style of this text will be modified'.dim);
console.log('The style of this text will be modified'.italic);
console.log('The style of this text will be modified'.underline);
console.log('The style of this text will be modified'.inverse);
console.log('The style of this text will be modified'.hidden);
console.log('The style of this text will be modified'.strikethrough);
但是, 如果你担心扩展String.prototype, 则可以使用非原型方法使用安全模式:
var colors = require('colors/safe');
console.log(colors.reset('The style of this text will be modified'));
console.log(colors.bold('The style of this text will be modified'));
console.log(colors.dim('The style of this text will be modified'));
console.log(colors.italic('The style of this text will be modified'));
console.log(colors.underline('The style of this text will be modified'));
console.log(colors.inverse('The style of this text will be modified'));
console.log(colors.hidden('The style of this text will be modified'));
console.log(colors.strikethrough('The style of this text will be modified'));
没有第三方库就做
如果你不想依赖任何模块, 则可以根据以下信息来实现自己的方法。
文字颜色
为了显示具有特定颜色的文本, 你需要将文本包装在特定的符号中, 控制台将对其进行解释。
要了解其工作原理, 请分析以下示例:
var myText = "Hello World";
var startWrapper = "[31m";
var closeWrapper = "[39m";
// Show some text in the console with red Color
console.log(startWrapper + myText + closeWrapper);
但是, 如果你在控制台中执行先前的代码, 它将无法正常工作!因为它将在控制台中输出纯文本” [31mHello World [39m]”。那么, 诀窍是什么呢?显示输出的console.log希望将纯字符串作为第一个参数(与Color.js的示例相同)。因此, 以下示例应工作:
// Show some text in the console with red Color
console.log("[31mHello World[39m");
下表指定了要显示的每种可用颜色的代码:
颜色 | 以。。开始 | 以。。结束 | 例子 |
---|---|---|---|
黑色 | [30m | [39m | [30mREPLACE-THIS [39m |
红色 | [31m | [39m | [31mREPLACE-THIS [39m |
绿色 | [32m | [39m | [32mREPLACE-THIS [39m |
黄色 | [33m | [39m | [33mREPLACE-THIS [39m |
蓝色 | [34m | [39m | [34mREPLACE-THIS [39m |
品红 | [35m | [39m | [35mREPLACE-THIS [39m |
青色 | [36m | [39m | [36mREPLACE-THIS [39m |
白色 | [37m | [39m | [37mREPLACE-THIS [39m |
灰色 | [90m | [39m | [90mREPLACE-THIS [39m |
背景颜色
为了显示具有特定背景颜色的文本, 你需要将文本包装在特定的符号内(即使它们已经在使用文本颜色), 并且控制台会对其进行解释。
背景颜色的工作方式与文本颜色相同。但是, 不是使用[3x前缀, 而是使用[4x。
颜色 | 以。。开始 | 以。。结束 | 例子 |
---|---|---|---|
黑色 | [40m | [49m | [40mREPLACE-THIS [49m |
红色 | [41m | [49m | [41mREPLACE-THIS [49m |
绿色 | [42m | [49m | [42mREPLACE-THIS [49m |
黄色 | [43m | [49m | [43mREPLACE-THIS [49m |
蓝色 | [44m | [49m | [44mREPLACE-THIS [49m |
品红 | [45m | [49m | [45mREPLACE-THIS [49m |
青色 | [46m | [49m | [46mREPLACE-THIS [49m |
白色 | [47m | [49m | [47mREPLACE-THIS [49m |
下面的示例应显示一些带有蓝色背景和白色文本颜色的文本:
console.log("[37m[44mOur Code World Rocks[49m[39m");
文字样式
为了将某些文本样式应用于文本, 你需要将文本包装在特定的符号内(在大多数情况下, 为了起作用, 它们必须已经具有文本颜色), 并且控制台会对其进行解释。
颜色 | 以。。开始 | 以。。结束 | 例子 |
---|---|---|---|
重启 | [0m | [0m | [0mREPLACE-THIS [0m |
胆大 | [1m | [22m | [1mREPLACE-THIS [22m |
暗淡 | [2m | [22m | [2mREPLACE-THIS [22m |
斜体 | [3m | [23m | [3mREPLACE-THIS [23m |
强调 | [4m | [24m | [4mREPLACE-THIS [24m |
逆 | [7m | [27m | [7mREPLACE-THIS [27m |
隐 | [8m | [28m | [8mREPLACE-THIS [28m |
删除线 | [9m | [29m | [9mREPLACE-THIS [29m |
下面的示例应显示一些带有下划线的红色文本:
console.log("[4m[31mSome text[39m[24m");
// Or in other order with the same result
console.log("[31m[4mSome text[24m[39m");
请注意, (显然)符号需要分别以它们的开始方式结束(<开始颜色标签| <打开样式标签| {某些文本} |关闭样式标签>关闭颜色标签>, 反之亦然)。
编码愉快!
评论前必须登录!
注册