本文概述
在Python中, 弦乐是表示Unicode字符的字节数组。但是, Python没有字符数据类型, 单个字符就是长度为1的字符串。方括号可用于访问字符串的元素。
创建一个字符串
Python中的字符串可以使用单引号, 双引号甚至三引号来创建。
# Python Program for
# Creation of String
# Creating a String
# with single Quotes
String1 = 'Welcome to the Geeks World'
print ( "String with the use of Single Quotes: " )
print (String1)
# Creating a String
# with double Quotes
String1 = "I'm a Geek"
print ( "\nString with the use of Double Quotes: " )
print (String1)
# Creating a String
# with triple Quotes
String1 = '''I'm a Geek and I live in a world of "Geeks"'''
print ( "\nString with the use of Triple Quotes: " )
print (String1)
# Creating String with triple
# Quotes allows multiple lines
String1 = '''Geeks
For
Life'''
print ( "\nCreating a multiline String: " )
print (String1)
输出如下:
String with the use of Single Quotes:
Welcome to the Geeks World
String with the use of Double Quotes:
I'm a Geek
String with the use of Triple Quotes:
I'm a Geek and I live in a world of "Geeks"
Creating a multiline String:
Geeks
For
Life
在Python中访问字符
在Python中, 可以使用索引方法来访问字符串的各个字符。索引允许负地址引用从字符串的后面访问字符, 例如-1表示最后一个字符, -2表示倒数第二个字符, 依此类推。
当访问索引超出范围时会导致IndexError。仅允许将整数作为索引, 浮点数或其他类型传递, 这将导致TypeError.
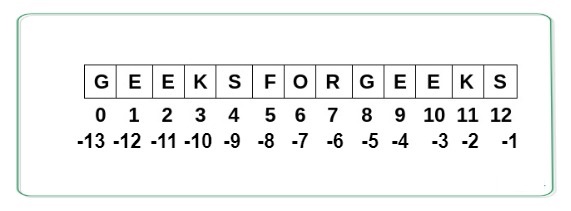
# Python Program to Access
# characters of String
String1 = "srcmini"
print ( "Initial String: " )
print (String1)
# Printing First character
print ( "\nFirst character of String is: " )
print (String1[ 0 ])
# Printing Last character
print ( "\nLast character of String is: " )
print (String1[ - 1 ])
输出如下:
Initial String:
srcmini
First character of String is:
G
Last character of String is:
s
字符串切片
要访问String中的一系列字符, 可以使用切片方法。通过使用Slicing运算符(冒号)完成对String的切片。
# Python Program to
# demonstrate String slicing
# Creating a String
String1 = "srcmini"
print ( "Initial String: " )
print (String1)
# Printing 3rd to 12th character
print ( "\nSlicing characters from 3-12: " )
print (String1[ 3 : 12 ])
# Printing characters between
# 3rd and 2nd last character
print ( "\nSlicing characters between " +
"3rd and 2nd last character: " )
print (String1[ 3 : - 2 ])
输出如下:
Initial String:
srcmini
Slicing characters from 3-12:
ksForGeek
Slicing characters between 3rd and 2nd last character:
ksForGee
从字符串删除/更新
在Python中, 不允许更新或删除字符串中的字符。这将导致错误, 因为不支持项目分配或从字符串中删除项目。尽管可以使用内置的del关键字删除整个String。这是因为字符串是不可变的, 因此一旦分配了字符串的元素就无法更改。只能将新字符串重新分配给相同的名称。
更新角色:
# Python Program to Update
# character of a String
String1 = "Hello, I'm a Geek"
print ( "Initial String: " )
print (String1)
# Updating a character
# of the String
String1[ 2 ] = 'p'
print ( "\nUpdating character at 2nd Index: " )
print (String1)
错误:
追溯(最近一次通话最近):String1 [2] =’p’TypeError中的文件” /home/360bb1830c83a918fc78aa8979195653.py”, 第10行, TypeError:” str”对象不支持项目分配
更新整个字符串:
# Python Program to Update
# entire String
String1 = "Hello, I'm a Geek"
print ( "Initial String: " )
print (String1)
# Updating a String
String1 = "Welcome to the Geek World"
print ( "\nUpdated String: " )
print (String1)
输出如下:
Initial String:
Hello, I'm a Geek
Updated String:
Welcome to the Geek World
删除字符:
# Python Program to Delete
# characters from a String
String1 = "Hello, I'm a Geek"
print ( "Initial String: " )
print (String1)
# Deleting a character
# of the String
del String1[ 2 ]
print ( "\nDeleting character at 2nd Index: " )
print (String1)
错误:
追溯(最近一次通话):文件” /home/499e96a61e19944e7e45b7a6e1276742.py”, 第10行, 位于del String1 [2]中TypeError:” str”对象不支持项目删除
删除整个字符串:
使用del关键字可以删除整个字符串。此外, 如果我们尝试打印字符串, 这将产生错误, 因为删除了字符串并且无法打印该字符串。
# Python Program to Delete
# entire String
String1 = "Hello, I'm a Geek"
print ( "Initial String: " )
print (String1)
# Deleting a String
# with the use of del
del String1
print ( "\nDeleting entire String: " )
print (String1)
错误:
追溯(最近一次通话):文件” /home/e4b8f2170f140da99d2fe57d9d8c6a94.py”, 行12(在print(String1)中)NameError:未定义名称” String1″
Python中的转义序列
在打印带有单引号和双引号的字符串时会导致
语法错误
因为字符串已经包含单引号和双引号, 因此不能使用这两个引号进行打印。因此, 要打印这样的字符串, 请使用三引号或转义序列来打印这样的字符串。
转义序列以反斜杠开头, 并且可以有不同的解释。如果使用单引号表示字符串, 则必须对字符串中存在的所有单引号进行转义, 并对双引号进行相同的操作。
# Python Program for
# Escape Sequencing
# of String
# Initial String
String1 = '''I'm a "Geek"'''
print ( "Initial String with use of Triple Quotes: " )
print (String1)
# Escaping Single Quote
String1 = 'I\'m a "Geek"'
print ( "\nEscaping Single Quote: " )
print (String1)
# Escaping Doule Quotes
String1 = "I'm a \"Geek\""
print ( "\nEscaping Double Quotes: " )
print (String1)
# Printing Paths with the
# use of Escape Sequences
String1 = "C:\\Python\\Geeks\\"
print ( "\nEscaping Backslashes: " )
print (String1)
输出如下:
Initial String with use of Triple Quotes:
I'm a "Geek"
Escaping Single Quote:
I'm a "Geek"
Escaping Double Quotes:
I'm a "Geek"
Escaping Backslashes:
C:\Python\Geeks\
要忽略字符串中的转义序列, [Ror[R表示字符串是原始字符串, 并且其中的转义序列将被忽略。
# Printing Geeks in HEX
String1 = "This is \x47\x65\x65\x6b\x73 in \x48\x45\x58"
print ( "\nPrinting in HEX with the use of Escape Sequences: " )
print (String1)
# Using raw String to
# ignore Escape Sequences
String1 = r "This is \x47\x65\x65\x6b\x73 in \x48\x45\x58"
print ( "\nPrinting Raw String in HEX Format: " )
print (String1)
输出如下:
Printing in HEX with the use of Escape Sequences:
This is Geeks in HEX
Printing Raw String in HEX Format:
This is \x47\x65\x65\x6b\x73 in \x48\x45\x58
字符串格式化
可以使用format()方法来格式化Python中的字符串, 该方法是用于格式化字符串的功能非常强大的工具。 String中的Format方法包含大括号{}作为占位符, 可以根据位置或关键字保存参数以指定顺序。
# Python Program for
# Formatting of Strings
# Default order
String1 = "{} {} {}" . format ( 'Geeks' , 'For' , 'Life' )
print ( "Print String in default order: " )
print (String1)
# Positional Formatting
String1 = "{1} {0} {2}" . format ( 'Geeks' , 'For' , 'Life' )
print ( "\nPrint String in Positional order: " )
print (String1)
# Keyword Formatting
String1 = "{l} {f} {g}" . format (g = 'Geeks' , f = 'For' , l = 'Life' )
print ( "\nPrint String in order of Keywords: " )
print (String1)
输出如下:
Print String in default order:
Geeks For Life
Print String in Positional order:
For Geeks Life
Print String in order of Keywords:
Life For Geeks
可以使用格式说明符将整数(例如二进制, 十六进制等)和浮点数四舍五入或以指数形式显示。
# Formatting of Integers
String1 = "{0:b}" . format ( 16 )
print ( "\nBinary representation of 16 is " )
print (String1)
# Formatting of Floats
String1 = "{0:e}" . format ( 165.6458 )
print ( "\nExponent representation of 165.6458 is " )
print (String1)
# Rounding off Integers
String1 = "{0:.2f}" . format ( 1 /6 )
print ( "\none-sixth is : " )
print (String1)
输出如下:
Binary representation of 16 is
10000
Exponent representation of 165.6458 is
1.656458e+02
one-sixth is :
0.17
可以使用格式说明符将字符串以left()或center(^)对齐, 并以冒号(:)分隔。
# String alignment
String1 = "|{:<10}|{:^10}|{:>10}|" . format ( 'Geeks' , 'for' , 'Geeks' )
print ( "\nLeft, center and right alignment with Formatting: " )
print (String1)
# To demonstrate aligning of spaces
String1 = "\n{0:^16} was founded in {1:<4}!" . format ( "srcmini" , 2009 )
print (String1)
输出如下:
Left, center and right alignment with Formatting:
|Geeks | for | Geeks|
srcmini was founded in 2009 !
旧样式的格式化是在不使用format方法的情况下进行的%操作符
# Python Program for
# Old Style Formatting
# of Integers
Integer1 = 12.3456789
print ( "Formatting in 3.2f format: " )
print ( 'The value of Integer1 is %3.2f' % Integer1)
print ( "\nFormatting in 3.4f format: " )
print ( 'The value of Integer1 is %3.4f' % Integer1)
输出如下:
Formatting in 3.2f format:
The value of Integer1 is 12.35
Formatting in 3.4f format:
The value of Integer1 is 12.3457
有用的字符串操作
- 字符串上的逻辑运算符
- 使用%的字符串格式
- 字符串模板类
- 分割字符串
- Python文档字符串
- 字符串切片
- 查找字符串中所有重复的字符
- Python中的反向字符串(5种不同方式)
- Python程序检查字符串是否是回文
字符串常量
内建函数 | 描述 |
---|---|
string.ascii_letters | ascii_lowercase和ascii_uppercase常数的串联。 |
string.ascii_lowercase | 小写字母的串联 |
string.ascii_uppercase | 大写字母的串联 |
string.digits | 字符串中的数字 |
string.hexdigits | 字符串中的十六进制 |
string.letters | 字符串小写和大写的串联 |
string.lowercase | 字符串必须包含小写字母。 |
string.octdigits | 字符串中的八位数字 |
string.punctuation | 具有标点符号的ASCII字符。 |
string.printable | 可打印的字符串 |
String.endswith() | 如果字符串以给定的后缀结尾, 则返回True;否则返回False。 |
String.startswith() | 如果字符串以给定前缀开头, 则返回True;否则返回False。 |
String.isdigit() | 如果字符串中的所有字符都是数字, 则返回” True”, 否则, 返回” False”。 |
String.isalpha() | 如果字符串中的所有字符均为字母, 则返回” True”;否则, 返回” False”。 |
string.isdecimal() | 如果字符串中的所有字符均为十进制, 则返回true。 |
str.format() | Python3中的一种字符串格式化方法, 它允许多次替换和值格式化。 |
String.index | 返回字符串中第一次出现的子字符串的位置 |
string.uppercase | 字符串必须包含大写字母。 |
string.whitespace | 一个字符串, 其中包含被视为空格的所有字符。 |
string.swapcase() | 方法将给定字符串的所有大写字符转换为小写, 反之亦然, 并将其返回 |
replace() | 返回字符串的副本, 其中所有出现的子字符串都将替换为另一个子字符串。 |
不推荐使用的字符串函数
内建函数 | 描述 |
---|---|
string.Isdecimal | 如果字符串中的所有字符均为十进制, 则返回true |
String.Isalnum | 如果给定字符串中的所有字符均为字母数字, 则返回true。 |
String.Isalnum | 如果字符串是标题大写的字符串, 则返回True |
String.partition | 在第一次出现分隔符时分割字符串, 并返回一个元组。 |
String.Isidentifier | 检查字符串是否为有效标识符。 |
String.len | 返回字符串的长度。 |
String.rindex | 如果找到子字符串, 则返回该字符串内子字符串的最高索引。 |
String.Max | 返回字符串中最高的字母字符。 |
String.min | 返回字符串中的最小字母字符。 |
String.splitlines | 返回字符串中的行列表。 |
string.capitalize | 返回第一个字符大写的单词。 |
string.expandtabs | 用字符串扩展制表符, 用一个或多个空格替换它们 |
string.find | 返回子字符串中的最低索引。 |
string.rfind | 找到最高的索引。 |
string.count | 返回字符串中子字符串sub的(不重叠)出现次数 |
string.lower | 返回s的副本, 但将大写字母转换为小写字母。 |
string.split | 如果没有可选的第二个参数sep或无, 则返回字符串的单词列表 |
string.rsplit() | 返回字符串s的单词列表, 从末尾扫描s。 |
rpartition() | 方法将给定的字符串分为三部分 |
string.splitfields | 仅与两个参数一起使用时, 返回字符串单词的列表。 |
string.join | 将单词的列表或元组与出现的sep连接起来。 |
string.strip() | 它返回删除前导和尾随字符的字符串的副本 |
string.lstrip | 返回删除了前导字符的字符串的副本。 |
string.rstrip | 返回删除了结尾字符的字符串副本。 |
string.swapcase | 将小写字母转换为大写字母, 反之亦然。 |
string.translate | 使用表格翻译字符 |
string.upper | 小写字母转换为大写字母。 |
string.ljust | 在给定宽度的字段中左对齐。 |
string.rjust | 在给定宽度的字段中右对齐。 |
string.center() | 在给定宽度的字段中居中对齐。 |
string-zfill | 在左边用零数字填充数字字符串, 直到达到给定的宽度。 |
string.replace | 返回字符串s的副本, 其中所有出现的子字符串old都替换为new。 |
string.casefold() | 返回小写的字符串, 可用于无大小写的比较。 |
string.encode | 将字符串编码为Python支持的任何编码。默认编码为utf-8。 |
string.maketrans | 返回可用于str.translate()的转换表 |
最近关于Python字符串的文章
有关Python字符串的更多视频:Python字符串方法–第2部分Python字符串方法–第3部分逻辑操作和字符串拆分
Python字符串程序
- 弦–套装1, 套装2
- 字符串方法–套装1, 套装2, 套装3
- 正则表达式(搜索, 匹配和查找全部)
- Python字符串标题方法
- 交换逗号和字符串中的点
- 程序将字符串转换为列表
- 计算并显示字符串中的元音
- Python程序检查密码的有效性
- Python程序使用给定字符串中的集合对元音数量进行计数
- 检查字符串中的URL
- 检查给定字符串中是否存在子字符串
- 检查两个字符串是否为字谜
- 用Python映射函数和Dictionary以求和ASCII值
- Python中的Map函数和Lambda表达式来替换字符
- Python中的SequenceMatcher, 用于最长的公共子字符串
- 完整打印带有姓氏的名字的首字母
- 从Python数据集中找到k个最常见的单词
- 从列表中查找输入字符串的所有紧密匹配项
- 检查二进制数中是否有K个连续的1
- Python中的Lambda和过滤器
- 在Python中使用不常见字符连接字符串
- 检查字符串的两半在Python中是否具有相同的字符集
- 在Python中找到字符串中的第一个重复单词
- Python序列中第二最重复的单词
- Python中的第K个非重复字符
- 在Python中反转给定字符串中的单词
- 在Python中使用逗号作为1000分隔符打印数字
- 使用pytrie模块在Python中进行前缀匹配
- Python Regex从字符串中提取最大数值
- 成对的两组完整字符串
- 从给定的句子中删除所有重复的单词
- 以升序对句子中的单词进行排序
- 反转句子中的每个单词
- Python代码按字母顺序打印两个字符串的公共字符
- Python程序拆分和连接字符串
- Python代码以单次遍历将空格移到字符串的开头
- 用Python运行游程编码
- 从Python中的给定字符串中删除所有重复项
- 在Python中增加字符的方法
- 使用pytrie模块在Python中进行前缀匹配
- 在Python中使用逗号作为1000分隔符打印数字
- 在Python中反转给定字符串中的单词
- 在Python中执行代码字符串
- Python中的字符串切片, 以检查是否可以通过递归删除将字符串变为空
- 在Python中打印转义字符的方法
- Python中的字符串切片以旋转字符串
- 计算字符串中单词的出现次数
- 从Python数据集中找到k个最常见的单词
- Python |完整打印带有姓氏的名字的首字母
- Python中的Zip函数更改为新的字符集
- Python String isnumeric()及其应用
- 在Python中按字典顺序对单词进行排序
- 使用Lambda表达式和reduce函数查找出现的奇数次
- Python字符串标题方法
- 以升序对句子中的单词进行排序
- 将字符列表转换为字符串
- Python groupby方法删除所有连续的重复项
- Python groupby方法删除所有连续的重复项
- 用于从字符串中删除第i个字符的Python程序
- 在Python中用数字替换字符串以进行数据分析
- Python中的格式化字符串文字(f字符串)
- Python文档字符串
- 使用内置函数排列给定的字符串
- 在Python中查找字符串中每个单词的频率
- 程序接受包含所有元音的字符串
- 计算一对字符串中的匹配字符数
- 以最大频率计数给定字符串中的所有前缀
- 程序检查字符串是否包含任何特殊字符
- 生成随机字符串, 直到生成给定的字符串
- Python程序无需使用内置函数即可计算大小写字符
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。
评论前必须登录!
注册